Postman Tips
Almost every software developer needs to work with an API at some point. I find it helpful to test API endpoints using Postman, a GUI which specializes in this. Some people prefer to just use curl, and that’s fine! But I find myself way more productive using postman, and find it worth the upfront investment to make things more efficient.1
Collections
The first time-saver would be to save requests that you are working with inside a Postman collection. Instead of having to remember the specific URL for a given API endpoint, and keep copy and pasting things, you can just select a request. This is what my current collection for Logikcull looks like:
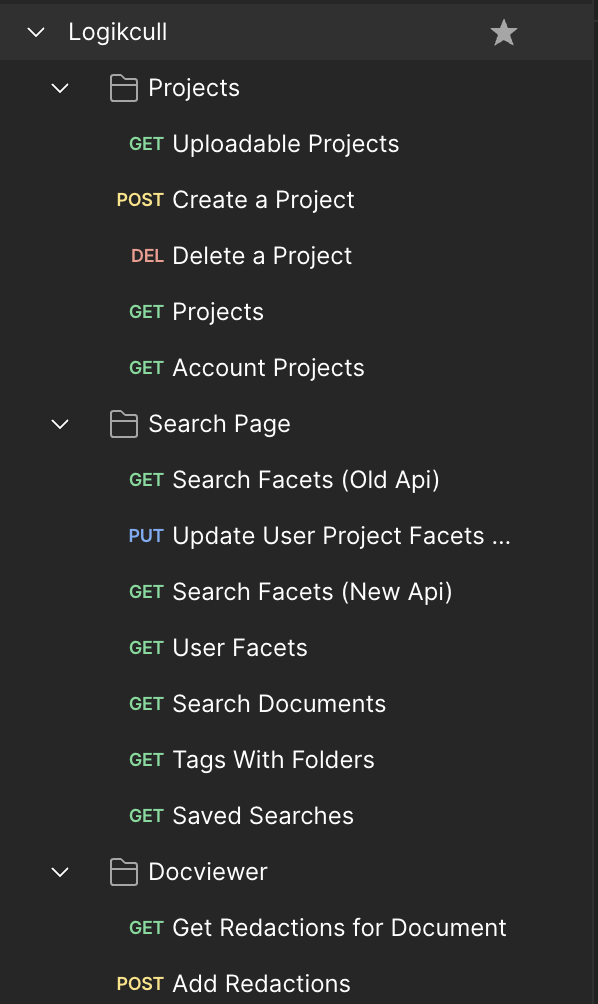
You can organize requests into folders, and clearly see the different HTTP verbs above.
Environments
It’s common for software devs to test multiple different environments - whether it’s dev, staging / QA, or prod (carefully that is!) For an API, you would probably have to change the base URL from something like api-dev
to api-staging1
or something. The easiest way to automate this is to setup different postman environments. For Logikcull, I setup 2 environments: one for dev, and the other for staging.
This is what my environment for dev looks like:
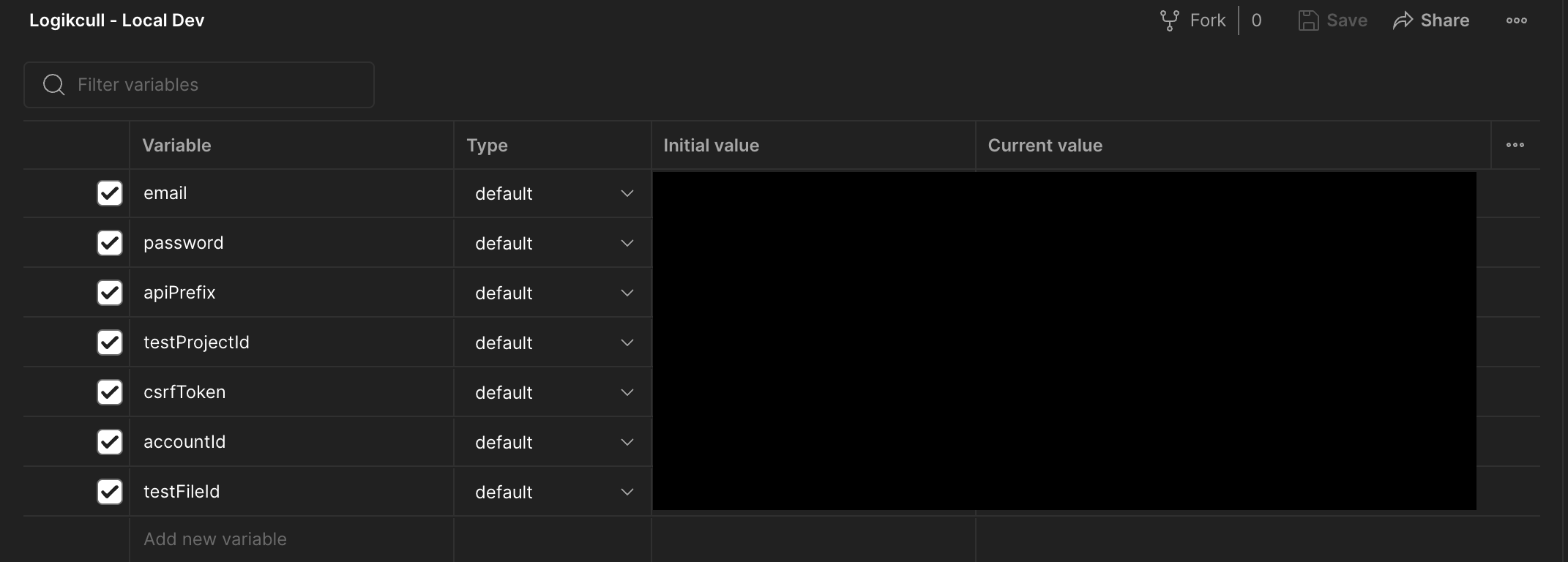
So in the simple case of having to switch the base URL, we can instead save each request referencing the environment variable of apiPrefix
, which looks like this:
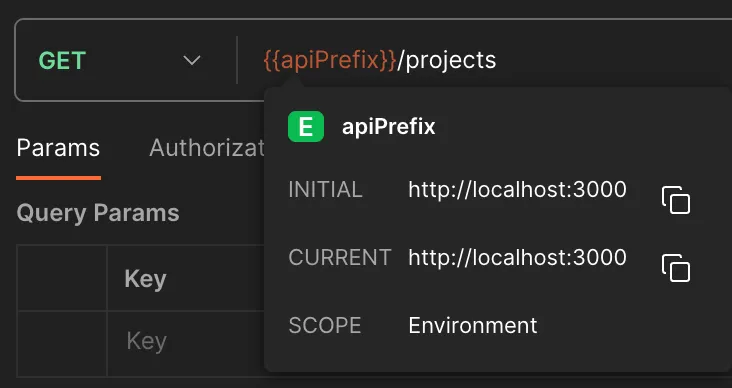
This means that the same request will work across environments without any changes needed - you just switch from the dev to staging environment in postman, and all of the env variables update! As you can see in the environment image above, I also use variables for things like email
/ password
(to easily login for different users across environments). It’s really handy for route params like userId
where you might have several different requests that reference the same user ID. Instead of needing to update all of the requests for a different user Id, you can just update the environment in one place!
Authentication
Any API will have authentication required. In the case of Logikcull, we have a fairly standard approach to this - using an HTTP cookie.
For the purposes of our end-to-end tests, we expose a /sessions
POST
route which can be called with a username / password, and the response includes the cookie and a CSRF token (used for PUT
/ POST
/ DELETE
requests).
Postman will automatically store cookies received from responses - so you just need to hit the authenticate route once, and then you’re all set. This is already a huge improvement in convenience vs always copying and pasting the cookie and including it via Curl.
However, in the case of Logikcull’s API, we still need to pass along the CSRF token as described above. This is where the real magic comes in! We first need to capture the CSRF token from the sessions
route. For this single request, we can leverage Postman tests to assert the token is included, and then save it.
pm.test('Get csrf token', () => {
// make sure request was successful
pm.response.to.have.status(201);
pm.response.to.be.json.withBody;
// extract csrf token from response body
const csrfToken = JSON.parse(responseBody).csrf_token;
pm.expect(csrfToken).to.exist;
// set csrf token into environment variable
pm.environment.set('csrfToken', csrfToken);
});
Okay - so now whenever the authenticate route is hit, we will save the csrfToken
into an environment variable. The real magic comes by using a postman pre-request script to include the CSRF token in subsequent requests. This can be isolated to the Logikcull collection, so you don’t pollute other API requests. The code for our situation looks like:
// fetch csrf token env variable
const csrfToken = pm.environment.get('csrfToken');
const requestMethod = pm.request.method.toUpperCase();
// only add the X-CSRF-Token if request is PUT / POST / DELETE
const methodsToAddHeader = ['POST', 'PUT', 'DELETE'];
if (methodsToAddHeader.includes(requestMethod)) {
pm.request.headers.add({ key: 'X-CSRF-token', value: csrfToken });
}
The pre-request script runs before any request in the logikcull collection - and will handle automatically adding the CSRF token to the X-CSRF-token
request header. Nice!
Collaboration on Teams
Collections can be shared across teams for easy collaboration on API development. Postman really seems to be upselling this to get more subscriptions to their service. For Logikcull, I exported the team collection with basic auth request and a couple others to a JSON file, which I uploaded to our internal documentation page. Devs can import the JSON and immediately start playing around with the API - which can make onboarding a smoother process.
Footnotes
-
This xkcd image seems relevant here:
I probably use Postman weekly on average (sometimes more frequently, sometimes less), and I would say the ideas in this post can easily shave 5 minutes off. So based on the graphic, it’s worth spending between 5 and 21 hours to make this more efficient. And luckily the things in this article take far shorter than that to start adding! ↩